
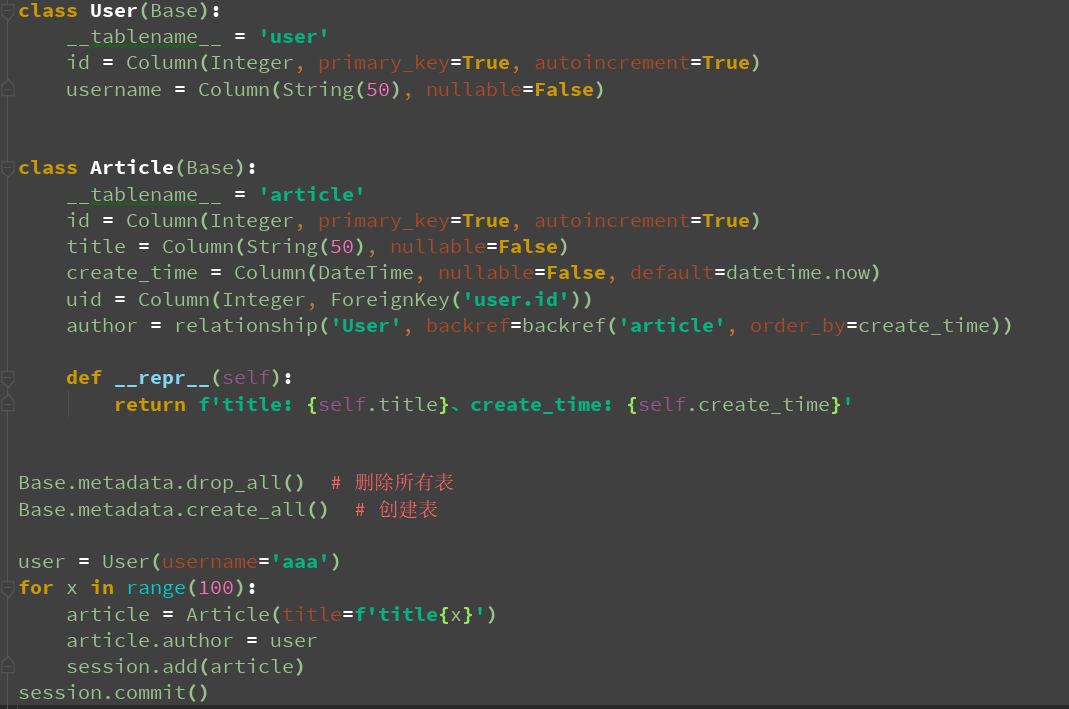
Return dialect. SQLAlchemy is a Python ORM (Object Relational Mapping) library that makes it easy to work with databases.

Uses Postgresql's UUID type, otherwise uses First, import the Python enum, the SQLAlchemy Enum, and your SQLAlchemy declarative base wherever you're going to declare your custom SQLAlchemy Enum column type. import uuidįrom sqlalchemy.types import TypeDecorator, BINARYįrom import UUID as psqlUUID Here is an approach based on the Backend agnostic GUID from the SQLAlchemy docs, but using a BINARY field to store the UUIDs in non-postgresql databases. The solution I came up with I was targeting MSSqlServer originally and then went MySql in the end, so I think my solution is a little more flexible as it seems to work fine on mysql and sqlite. If it doesn't, you would likely get errors when the table is created. Unless I'm missing something the above solution will work if the underlying database has a UUID type. I welcome any suggestions for improving it. See the section Dialects for information on the various backends available.
#Sqlalchemy sqlite install#
Dialects for the most common databases are included with SQLAlchemy a handful of others require an additional install of a separate dialect. Leave a comment if you see a glaring error with it. SQLAlchemy includes many Dialect implementations for various backends. Other than that everything seems to work fine, and so I'm throwing it out there.
#Sqlalchemy sqlite full#
SQLAlchemy provides a full suite of well known enterprise-level persistence patterns, designed for efficient and high-performing database access, adapted into a simple and Pythonic domain language. One disadvantage I've found is that at least in phpymyadmin, you can't edit records because it implicitly tries to do some sort of character conversion for the "select * from table where id =." and there's miscellaneous display issues. SQLAlchemy is the Python SQL toolkit and Object Relational Mapper that gives application developers the full power and flexibility of SQL. I believe storing as binary(16 bytes) should end up being more efficient than the string representation(36 bytes?), And there seems to be some indication that indexing 16 byte blocks should be more efficient in mysql than strings. Return Column(id_column_name,UUID(),primary_key=True,default=uuid.uuid4) Raise ValueError,'value %s is not a valid uuid.UUID' % valueĭef process_result_value(self,value,dialect=None): If value and isinstance(value,uuid.UUID):Įlif value and not isinstance(value,uuid.UUID): Types.TypeDecorator._init_(self,length=)ĭef process_bind_param(self,value,dialect=None): from sqlalchemy import typesįrom import MSBinary I've been refining a UUID column type for the past few months and I think I've finally got it solid. SQLAlchemy allows you to construct queries with Python functions.
#Sqlalchemy sqlite how to#
I think it has some advantages over autoincrement that make it worth it. Learn how to define a database schema and query an SQLite database with SQLAlchemy. Regardless of how my colleagues who really care about proper database design feel about UUID's and GUIDs used for key fields. I wrote this and the domain is gone but here's the guts.
